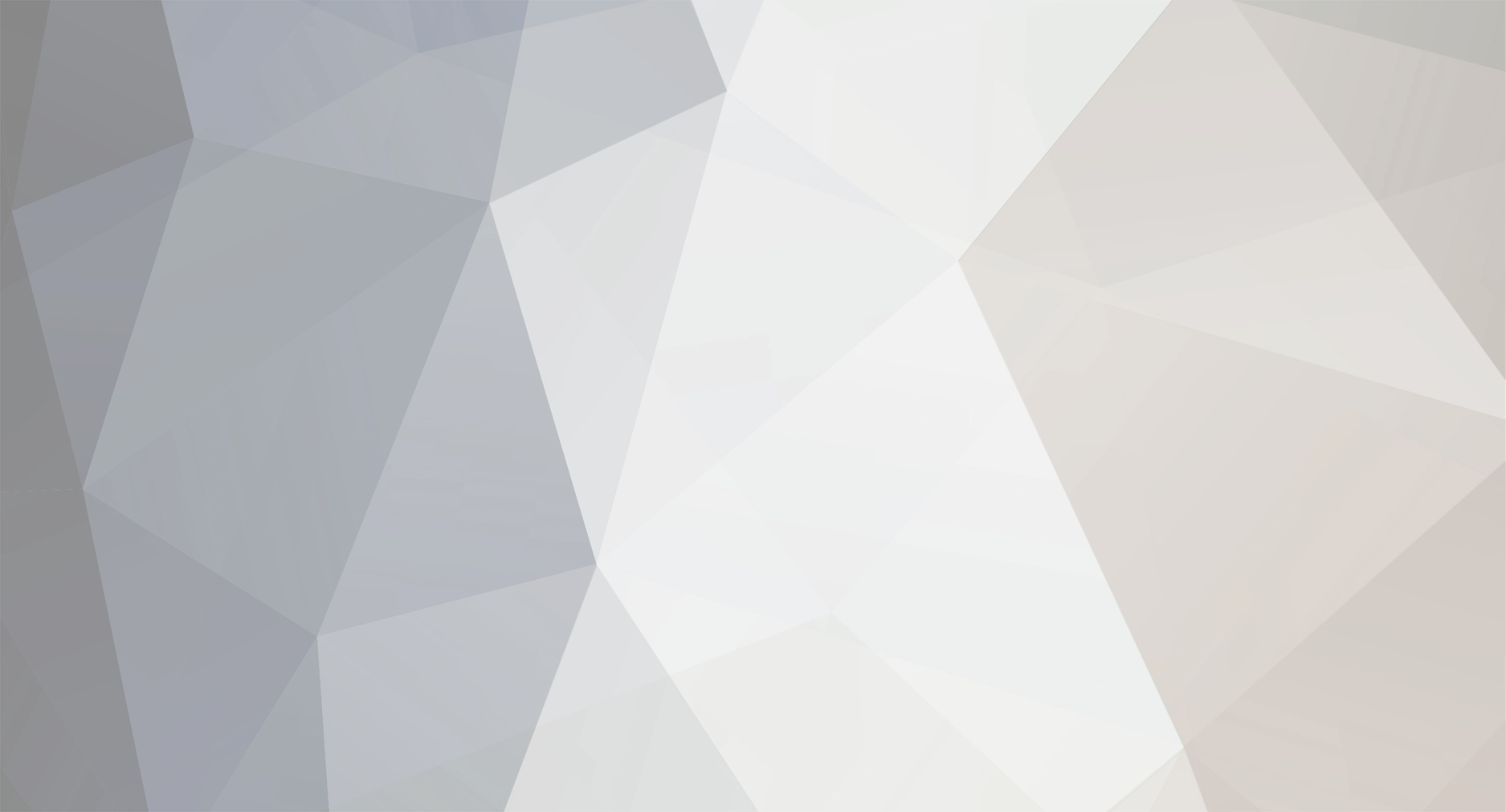
PACI
-
Contador contenido
501 -
Ingreso
-
Última visita
-
Días ganados
58
Mensajes publicados por PACI
-
-
-
- Este es un tema popular.
- Este es un tema popular.
-
PyObject * grpSaveScreenShotToPath(PyObject * poSelf, PyObject * poArgs) { char * szBasePath; if (!PyTuple_GetString(poArgs, 0, &szBasePath)) return Py_BuildException(); struct tm * tmNow; time_t ct; ct = time(0); tmNow = localtime(&ct); char szPath[MAX_PATH + 256]; snprintf(szPath, sizeof(szPath), "%s%02d%02d_%02d%02d%02d.jpg", szBasePath, tmNow->tm_mon + 1, tmNow->tm_mday, tmNow->tm_hour, tmNow->tm_min, tmNow->tm_sec); BOOL bResult = CPythonGraphic::Instance().SaveScreenShot(szPath); return Py_BuildValue("(is)", bResult, szPath); } //////////////////////////////////////////////////////////////////////////////// bool CPythonGraphic::SaveScreenShot(const char * c_pszFileName) { HRESULT hr; LPDIRECT3DSURFACE8 lpSurface; D3DSURFACE_DESC stSurfaceDesc; if (FAILED(hr = ms_lpd3dDevice->GetBackBuffer(0, D3DBACKBUFFER_TYPE_MONO, &lpSurface))) { TraceError("Failed to get back buffer (0x%08x)", hr); return false; } if (FAILED(hr = lpSurface->GetDesc(&stSurfaceDesc))) { TraceError("Failed to get surface desc (0x%08x)", hr); SAFE_RELEASE(lpSurface); return false; } UINT uWidth = stSurfaceDesc.Width; UINT uHeight = stSurfaceDesc.Height; switch( stSurfaceDesc.Format ) { case D3DFMT_R8G8B8 : case D3DFMT_A8R8G8B8 : case D3DFMT_X8R8G8B8 : case D3DFMT_R5G6B5 : case D3DFMT_X1R5G5B5 : case D3DFMT_A1R5G5B5 : break; case D3DFMT_A4R4G4B4 : case D3DFMT_R3G3B2 : case D3DFMT_A8R3G3B2 : case D3DFMT_X4R4G4B4 : case D3DFMT_A2B10G10R10 : TraceError("Unsupported BackBuffer Format(%d). Please contact Metin 2 Administrator.", stSurfaceDesc.Format); SAFE_RELEASE(lpSurface); return false; } D3DLOCKED_RECT lockRect; if (FAILED(hr = lpSurface->LockRect(&lockRect, NULL, D3DLOCK_NO_DIRTY_UPDATE | D3DLOCK_READONLY | D3DLOCK_NOSYSLOCK))) { TraceError("Failed to lock the surface (0x%08x)", hr); SAFE_RELEASE(lpSurface); return false; } BYTE* pbyBuffer = new BYTE[uWidth * uHeight * 3]; if (pbyBuffer == NULL) { lpSurface->UnlockRect(); lpSurface->Release(); lpSurface = NULL; TraceError("Failed to allocate screenshot buffer"); return false; } BYTE* pbySource = (BYTE*) lockRect.pBits; BYTE* pbyDestination = (BYTE*) pbyBuffer; for(UINT y = 0; y < uHeight; ++y) { BYTE *pRow = pbySource; switch( stSurfaceDesc.Format ) { case D3DFMT_R8G8B8 : for(UINT x = 0; x < uWidth; ++x) { *pbyDestination++ = pRow[2]; // Blue *pbyDestination++ = pRow[1]; // Green *pbyDestination++ = pRow[0]; // Red pRow += 3; } break; case D3DFMT_A8R8G8B8 : case D3DFMT_X8R8G8B8 : for(UINT x = 0; x < uWidth; ++x) { *pbyDestination++ = pRow[2]; // Blue *pbyDestination++ = pRow[1]; // Green *pbyDestination++ = pRow[0]; // Red pRow += 4; } break; case D3DFMT_R5G6B5 : { for(UINT x = 0; x < uWidth; ++x) { UINT uColor = *((UINT *) pRow); BYTE byBlue = (uColor >> 11) & 0x1F; BYTE byGreen = (uColor >> 5) & 0x3F; BYTE byRed = uColor & 0x1F; *pbyDestination++ = (byBlue << 3) | (byBlue >> 2); // Blue *pbyDestination++ = (byGreen << 2) | (byGreen >> 2); // Green *pbyDestination++ = (byRed << 3) | (byRed >> 2); // Red pRow += 2; } } break; case D3DFMT_X1R5G5B5 : case D3DFMT_A1R5G5B5 : { for(UINT x = 0; x < uWidth; ++x) { UINT uColor = *((UINT *) pRow); BYTE byBlue = (uColor >> 10) & 0x1F; BYTE byGreen = (uColor >> 5) & 0x1F; BYTE byRed = uColor & 0x1F; *pbyDestination++ = (byBlue << 3) | (byBlue >> 2); // Blue *pbyDestination++ = (byGreen << 3) | (byGreen >> 2); // Green *pbyDestination++ = (byRed << 3) | (byRed >> 2); // Red pRow += 2; } } break; } // increase by one line pbySource += lockRect.Pitch; } if(lpSurface) { lpSurface->UnlockRect(); lpSurface->Release(); lpSurface = NULL; } bool bSaved = SaveJPEG(c_pszFileName, pbyBuffer, uWidth, uHeight); if(pbyBuffer) { delete [] pbyBuffer; pbyBuffer = NULL; } if(bSaved == false) { TraceError("Failed to save JPEG file. (%s, %d, %d)", c_pszFileName, uWidth, uHeight); return false; } if (g_isScreenShotKey) { FILE* srcFilePtr = fopen(c_pszFileName, "rb"); if (srcFilePtr) { fseek(srcFilePtr, 0, SEEK_END); size_t fileSize = ftell(srcFilePtr); fseek(srcFilePtr, 0, SEEK_SET); char head[21]; size_t tailSize = fileSize - sizeof(head); char* tail = (char*)malloc(tailSize); fread(head, sizeof(head), 1, srcFilePtr); fread(tail, tailSize, 1, srcFilePtr); fclose(srcFilePtr); char imgDesc[64]; GenScreenShotTag(c_pszFileName, GetCRC32(tail, tailSize), imgDesc, sizeof(imgDesc)); int imgDescLen = strlen(imgDesc) + 1; unsigned char exifHeader[] = { 0xe1, 0, // blockLen[1], 0, // blockLen[0], 0x45, 0x78, 0x69, 0x66, 0x0, 0x0, 0x49, 0x49, 0x2a, 0x0, 0x8, 0x0, 0x0, 0x0, 0x1, 0x0, 0xe, 0x1, 0x2, 0x0, imgDescLen, // textLen[0], 0, // textLen[1], 0, // textLen[2], 0, // textLen[3], 0x1a, 0x0, 0x0, 0x0, 0x0, 0x0, 0x0, 0x0, }; exifHeader[2] = sizeof(exifHeader) + imgDescLen; FILE* dstFilePtr = fopen(c_pszFileName, "wb"); //FILE* dstFilePtr = fopen("temp.jpg", "wb"); if (dstFilePtr) { fwrite(head, sizeof(head), 1, dstFilePtr); fwrite(exifHeader, sizeof(exifHeader), 1, dstFilePtr); fwrite(imgDesc, imgDescLen, 1, dstFilePtr); fputc(0x00, dstFilePtr); fputc(0xff, dstFilePtr); fwrite(tail, tailSize, 1, dstFilePtr); fclose(dstFilePtr); } free(tail); } } return true; }
puede que el syserr.txt te diga algo de utilidad.
-
-
Te dejo esta versión que he realizado asi rapido
---------------------------------- -- Pedido Metin2Zone -- Creado por Elite™ ---------------------------------- quest miquest begin state start begin -- En idnpc pon el id when idnpc.chat."Transformarme" begin say_title(npc.get_race()) --Yang a pagar local yang = 10000 --Nivel minimo local nivel = 100 if pc.level < nivel and pc.gold < yang then say("Para transformate necesitas ser almenos nivel 100") say("ademas de tener "..yang.." Yang.") else local monstruos = { -- Tabla con los Mobs {101,"Perro Salvaje"}, {102,"Lobo"}, {103,"Lobo Alfa"}, {104,"Lobo Azul"}, {105,"Lobo Alfa Azul"}, {106,"Lobo Gris"}, {107,"Lobo Alfa Gris"}, {108,"Jabali"}, {109,"Jabali Rojo"}, {110,"Oso"}, {111,"Oso Pardo"}, {112,"Oso Negro"}, {113,"Oso Marron"}, {114,"Tigre"}, } local menu = {} say("En que mostruo quieres transformate") for id,valor in ipairs(monstruos) do table.insert(menu, valor[2]) end table.insert(menu, "Cancelar") local escoge = select_table(menu) if escoge != table.getn(menu) then --En tiempo ponerlo en segundos pc.polymorph(monstruos[escoge][1],tiempo) pc.changegold(-yang) end end end end end
En la tabla debes añadir todos los mobs que quieras, asi el usuario elegira por el nombre y asi no debe saberse el id del monstruo
local monstruos = {101, 102, 103, ..., 114} local menu = {} for _, vnum in ipairs(monstruos) do table.insert(menu, mob_name(vnum)) end table.insert(menu, "Cancelar") (...) pc.polymorph(monstruos[escoge])
pc.level y pc.gold no son seguros. Recomiendo pc.get_level() y pc.get_gold().
- autodesk_metin2 reacciono a esto
-
1
-
-
locale_game o locale_interface.txt
- angeluchiha18 reacciono a esto
-
1
-
Cliente.
-
uiToolTip.ToolTip.AddItemData
item.ITEM_TYPE_METIN
-
-
-
Hola.
No se de Java, pero se parece a C++.
Existen diferentes data-types en programación, cada uno puede almacenar una cantidad diferente de memoria.
int es un numero entero (0 - 2M)
double es un numero decimal, al igual que float, pero con la diferencia de que, tal como su nombre indica, puede almacenar el doble. Mientras float puede almacenar un numero decimal de 7 dígitos, double lo hace con 15.
La palabra static se puede utilizar para una variable, una función, o una clase. Una vez que declares una variable estática, esta permanecerá en la memoria y aunque la inicies varias veces, su valor no cambiará, por que ya la iniciaste una vez. Ejemplo:
for (int i = 0; i < 5; i++){ static int c = 0; c++; }
En este for-loop, dentro de el se declara la variable estática c (que es un integer) y se le suma siempre 1. Cuando i == 0, se guardará esa variable en la memoria y se le sumará 1, quedará c == 1. El caso es que cuando i > 0, esta variable seguirá teniendo el mismo valor, y no se reiniciará. No sé si me explico bien.
Sobre los void. Son un tipo de función que no devuelven nada. Mientras un int tiene que devolver un int, y un long un long, void no tiene ningún tipo de return.
String[] args, son los argumentos que la función main() puede tomar, todos ellos guardados en un array de tipo string.
Si utilizas esto, tu función main() podrá tener todos los argumentos que quieras, y los llamas mediante el array args y el index del argumento. Como por ejemplo:
main("Primer Argumento", "Segundo Argumento"); ---- int main(String[] args){ System.out.printIn(args[0]); // Primer Argumento System.out.printin(args[1]); // Segundo Argumento return 0; }
La ultima pregunta no te la puedo responder porque no se, pero creo que con una búsqueda rápida encontraras tu respuesta.
-
Esta muy bien, pero solo un par de cosillas:
- Puedes utilizar la funcion item_name(itemVnum) para los nombres de los objetos, no hay necesidad de añadir ese dato a la tabla.
- También es posible utilizar la función npc.get_race() para obtener el vnum del NPC seleccionado, de este modo no se necesita cambiarlo en el mob_name().
-
Entonces ese ID es erróneo.
-
-
no esta mal me gusta tu idea posiblemente si tengo tiempo ayude en algunos cursos aunque creo que no es el mejor foro para esto
Este foro le ha dado muchas cosas a muchos de nosotros, pena que solo 4 o 5 sigamos aquí.
-
Looks great.
-
-
-
Update:
- Si se desconecta dentro de la dungeon y el grupo sigue en ella, el jugador puede volver a unirse a los compañeros (dura 5 minutos);
- Los Ochao Healers ahora curan al Jotun Thrym (sin efecto, todavía).
-
Notas:
- El En-Tai Guardian es invocado cuando se mata al Ochao Bodyguard, e sale en unas coordenadas aleatorias.
- El Portal de entrada al Bosque tiene X minutos de spawn (por defecto, 1). Después de ese tiempo, desaparece;
- Se necesita un Grupo de Y miembros para entrar (por defecto 5, se puede remover esta comprobación, tal como se hizo en el vídeo);
- Todos los miembros del Grupo deben poseer un item de entrada (se puede remover esta comprobación);
- Cuando se sale de la dungeon, hay un delay de Z minutos/horas para volver a entrar (se puede quitar, editar).
-
¿Entonces son 50€ para aprender a hacer MOBs desde 0, como tú, o son 50€ por aprender a sacar cosas del Metin2.es?
¿Qué enseña el cursor?
- (...) extraer los mobs de juegos MMORPG (...).
- (...) adaptador (...) transformarlos a Metin2
- Adaptar (...).
-
Se ha bajado el precio de la dungeon:
El precio base es de 60€ (euros), y el método de pago es Paypal.
-
Lo que pasa es que no has cerrado el when login, estás intentando poner un when, dentro de otro when, y eso no se puede.
Esta parte:
when login with pc.get_level()>=5 or pc.get_level()>=5 begin set_state("La_formacion") when letter begin
Tiene que quedar así:
when login with pc.get_level()>=5 or pc.get_level()>=5 begin set_state("La_formacion") end when letter begin
[Venta] Mis sistemas
en Servicios y compraventa
Publicado
ola soi conprador suyo i la nueva lib me a borrado los fyles.